While Loop
Contents
4.1. While Loop#
The while loop is a control flow statement that allows instructions/statements/code to be executed repeatedly based on a given condition.
4.1.1. What is a while loop?#
The syntax of the while loop is as follows:
while (<condition>) {
<statements>;
}
<other statements>;
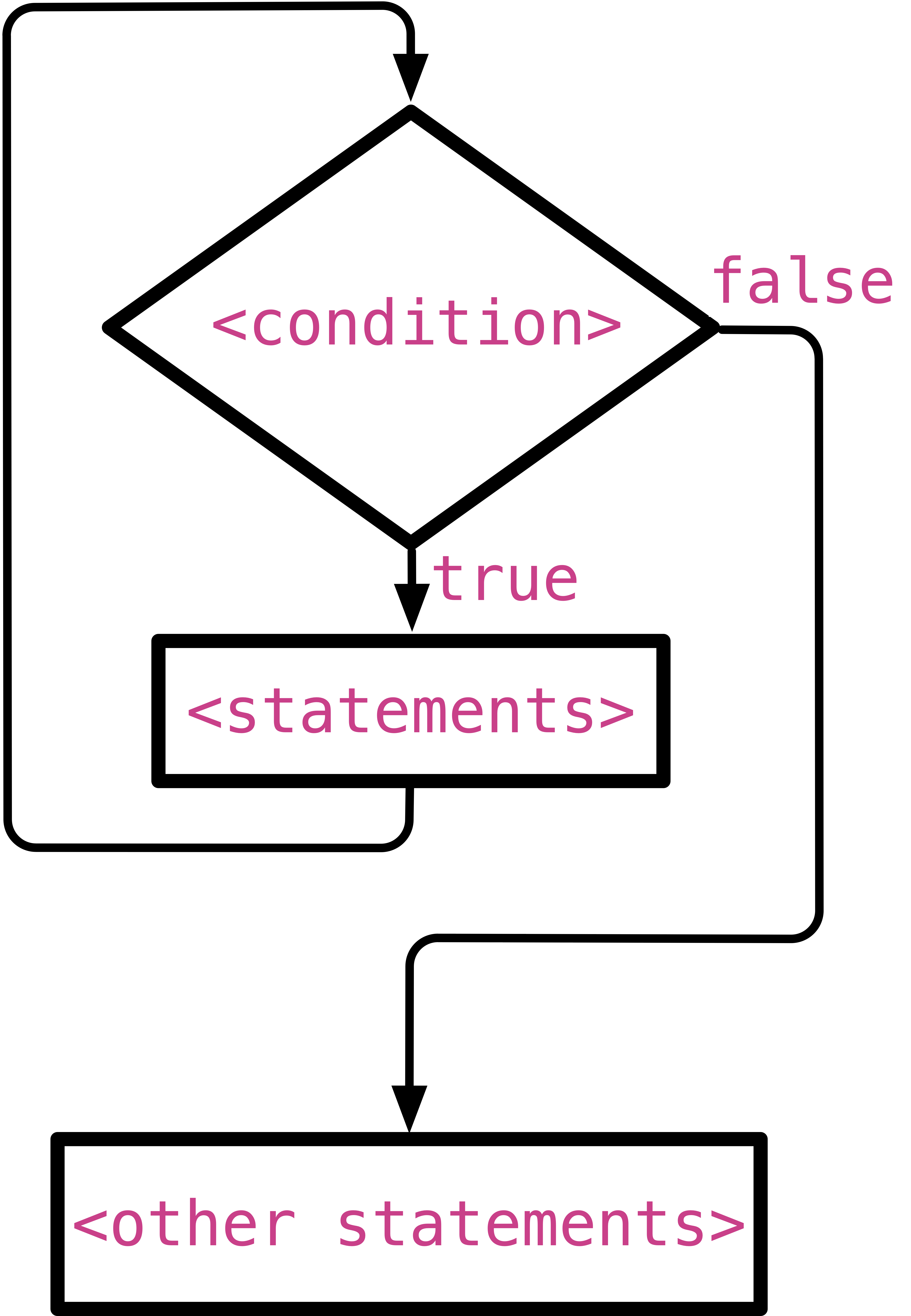
Fig. 4.1 The flow chart of a while loop.#
As the above flow chart shows, the execution of the while loop starts by:
Checking the condition of the loop.
If the condition is
true
, the statements inside the curly braces will be executed.Repeat 1 and 2 until the condition becomes
false
.Once the condition becomes
false
, the while loop will exit and nothing inside the curly braces will be executed anymore. The program will continue executing the statements after the while loop.
For example, the following program will print out the numbers \(1\) through \(3\).

Fig. 4.2 The execution of a program with a while loop.#
Output
1 2 3
To print numbers \(1\) to \(10\), we can change the condition to i <= 10
. The following program will print out the numbers \(1\) through \(10\). Download while-print-nums.c
if you want to run the program yourself.
Code
#include <stdio.h>
int main(void) { int i = 1; while (i <= 10) { printf("%d ", i); i++; } return 0; }
Exercise
Write a C program that takes in from the user numbers and calculates the sum of the numbers. The program should stop when the user enters a negative number.
Step 1: Toy example. For example, if the user enters 18, 5, 3, 2, 1, -1, the program should print out the sum of the numbers \(18 + 5 + 2 + 1\), which is 29.
Step 2: Think of a solution! The program should repeatedly take in numbers from the user. Repetition needs a loop. Repetition is for:
repeatedly entering numbers from the user
repeatedly adding the entered number to the sum.
This is on one condition, if the numbers were positive. The program should stop when the user enters a negative number.
Step 3: Decompose into steps. Writing the steps down requires us to write a pseudocode. Pseudocode is an informal way of writing code that helps programmers develop code without worrying about syntax or details. Pseudocode is a good way to think about the steps that the program should take.
A potential pseudocode for this program is as follows:
Initialize the sum to zero.
Take in a number from the user.
Check if the number is negative. If the number is negative, exit the while loop.
If the number is not negative, add the number to the sum.
Repeat steps 2-4 until the user enters a negative number.
Step 4: Draw your solution. The following flow chart shows the steps that the program should take.
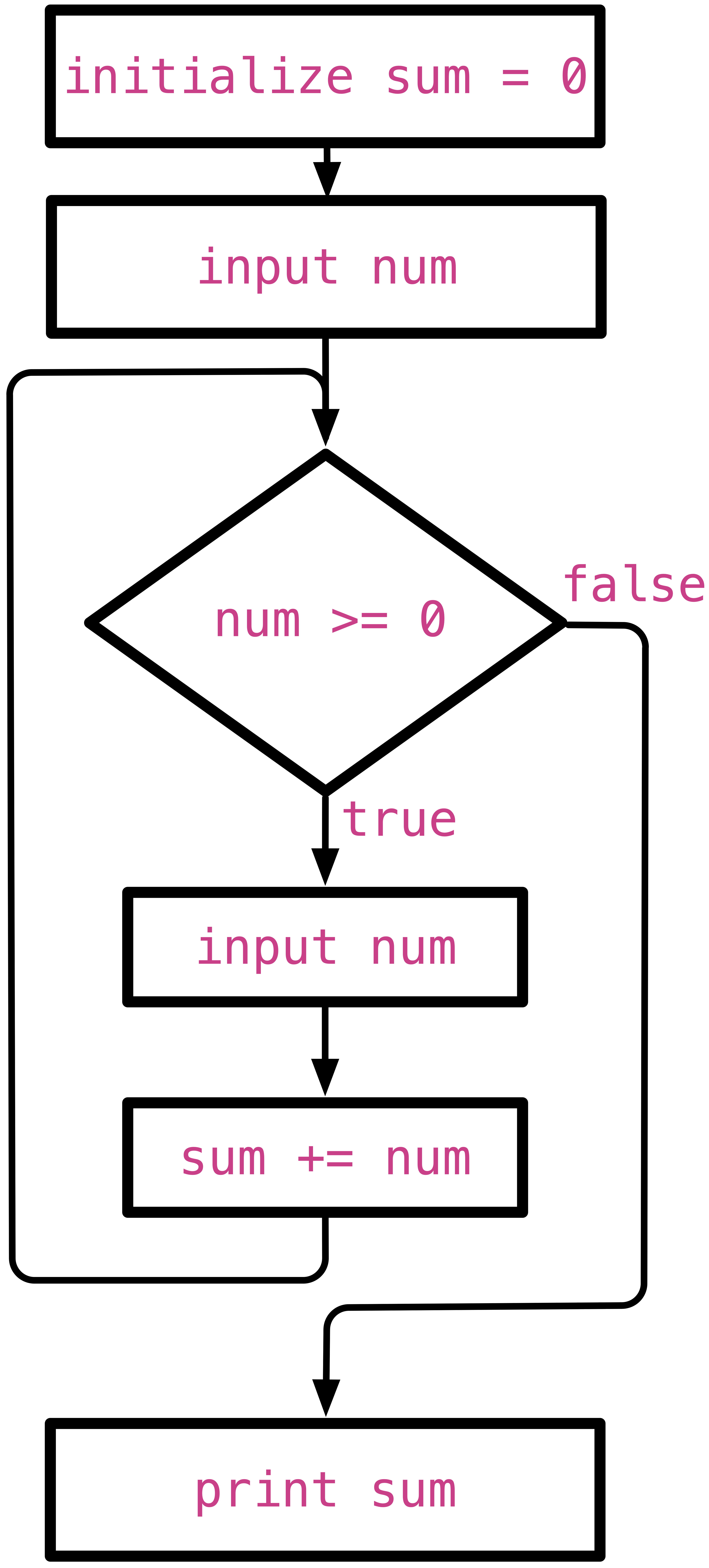
Fig. 4.3 The flow chart of the while loop exercise that finds the sum of numbers entered by the user until the user enters a negative number.#
Step 5: Write the code. Download sum-numbers-while.c
to get the following code.
#include <stdio.h>
int main(void){ int sum = 0; int num; printf("Enter a number: "); scanf("%d", &num); while(num >= 0){ sum += num; scanf("%d", &num); } printf("The sum is %d\n", sum); return 0; }
Common Confusions!
Should we take the number from the user inside or outside the while loop? Outside the while loop. This is because we need to check if the number is negative before we add it to the sum. If we decide to take in the first number from the user inside the while loop, we would have already passed the condition of the while loop.
Should we initialize the sum
to zero? Yes, we should initialize the sum
to zero. This is because the sum
should be zero if the user enters a negative number in the beginning. If we do not initialize the sum
to zero, the sum
will be undefined.
Step 6: Test your code. Test your code with other numbers. For example, try entering a negative number first. What happens? The sum should be 0. Try entering a zero number. What happens? The while loop should not stop and you should be still able to enter numbers.
Kindly, refer to the following video if you want to trace the code above.
4.1.2. Infinite Loops#
What happens when the condition in the while loop is always true? The while loop will never stop and the program will never exit. This is called an infinite loop. For example, the following program will never stop since i > 0
is always true
:
Code
#include <stdio.h>
int main(void){ int i = 1; while(i > 0){ //will always be true printf("%d ", i); i++; } return 0; }
Quiz
0 Questions