For loops
Contents
4.3. For loops#
In the previous sections, we discussed how to repeat instructions using while and do-while loops. In this section, we will discuss the for loop.
4.3.1. Forming the structure of a for loop from a while loop#
Recall the example, where we used while and do-while loops to print numbers between \(1\) and \(3\). In the following code, we highlight the specific structure of the while loop that allows us to go through fixed number of iterations. The structure highlights three features: initialization, condition, and increment.
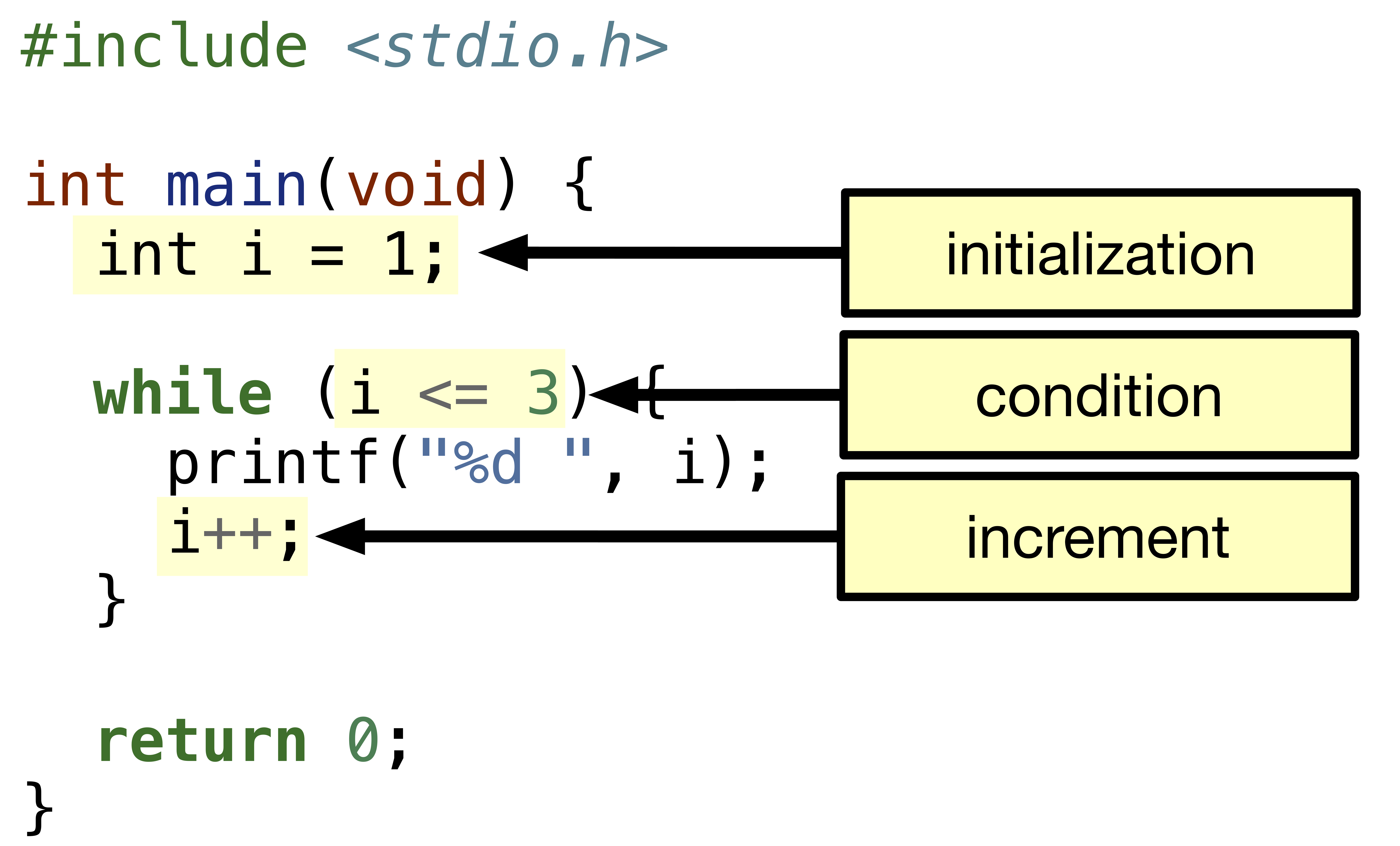
Fig. 4.7 The main features of while loop for fixed number of iterations: initialization, condition, and increment.#
The pre-mentioned structure is common, so C has a special loop called the for loop that allows us to write the same code in a more compact way. The syntax of the for loop is as follows:
for (<initialization>;<condition>;<increment>) {
<statements>;
}
<other statements>;
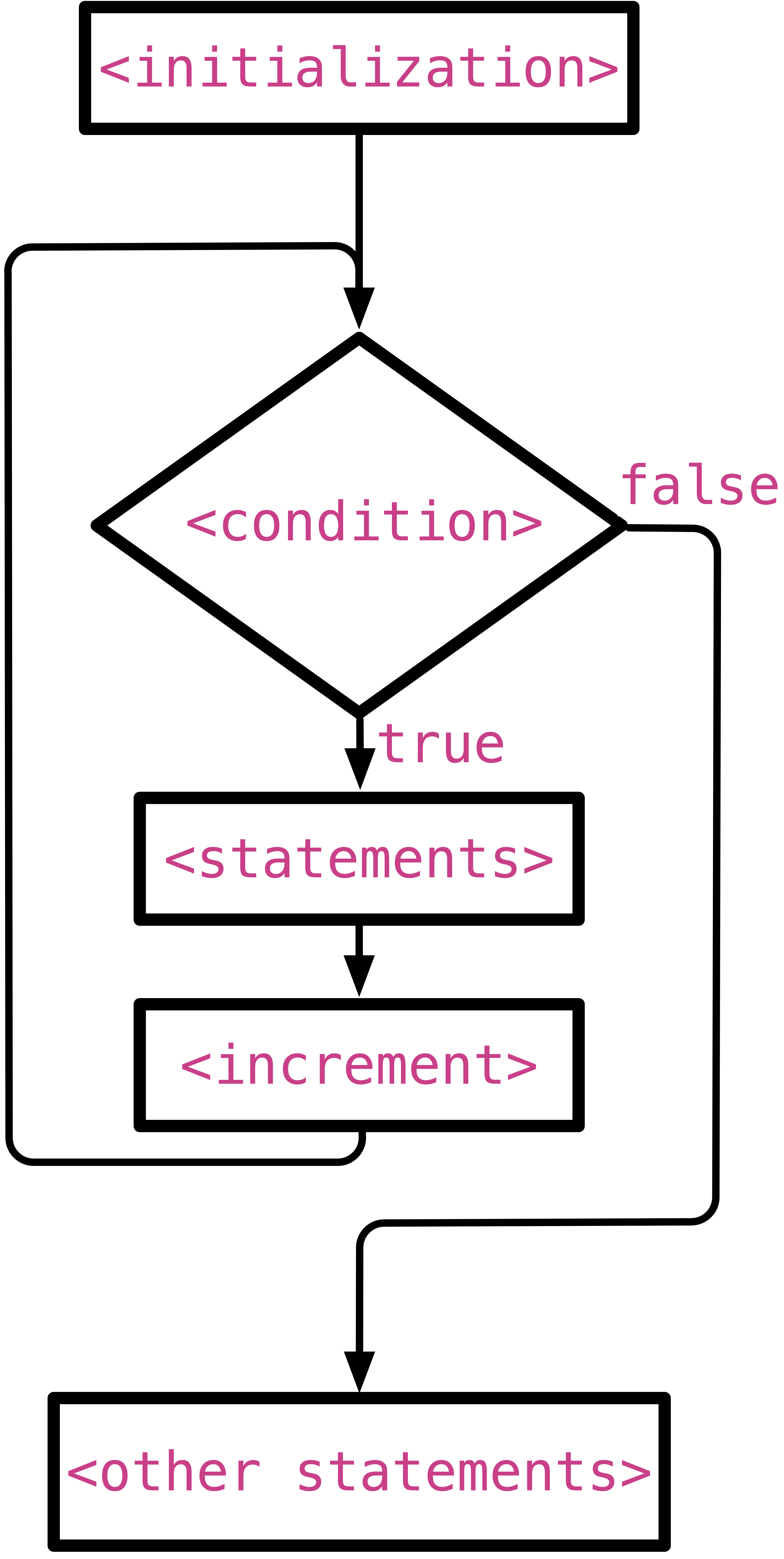
Fig. 4.8 The flow chart of a for loop.#
The following figure shows the order of execution in a for loop that prints numbers from \(1\) to \(3\).

Fig. 4.9 The execution of a program with a for loop.#
Again if we want to print numbers from \(1\) to \(10\), we only change the condition from i <= 3
to i <= 10
. The following program will print out the numbers \(1\) through \(10\) using a for loop. Download for-print-nums.c
if you want to run the program yourself.
Code
#include <stdio.h>
int main(void) { for (int i = 1; i <= 10; i++) { printf("%d ", i); } return 0; }
4.3.2. Scope of the loop variable#
In the for
loop, the initialization and declaration of the loop variable are done in the same statement, e.g., int i = 1
. This makes the i
variable only visible inside the for loop. After the for loop, the loop variable is no longer visible.
For example, if we compile the following code, we would get a compilation error.
Code with compilation error
#include <stdio.h>
int main(void) { for (int i = 1; i <= 10; i++) { // declare & initialize the loop variable inside the loop printf("%d ", i); }
printf("\nWe exited the loop with i = %d \n", i); return 0; }
Error
The error in the following figure is because the loop variable i
is not visible outside the loop. We refer to the visibility of the loop variable as the scope of the loop variable. i
is out of scope after the loop. We will discuss scope more later!
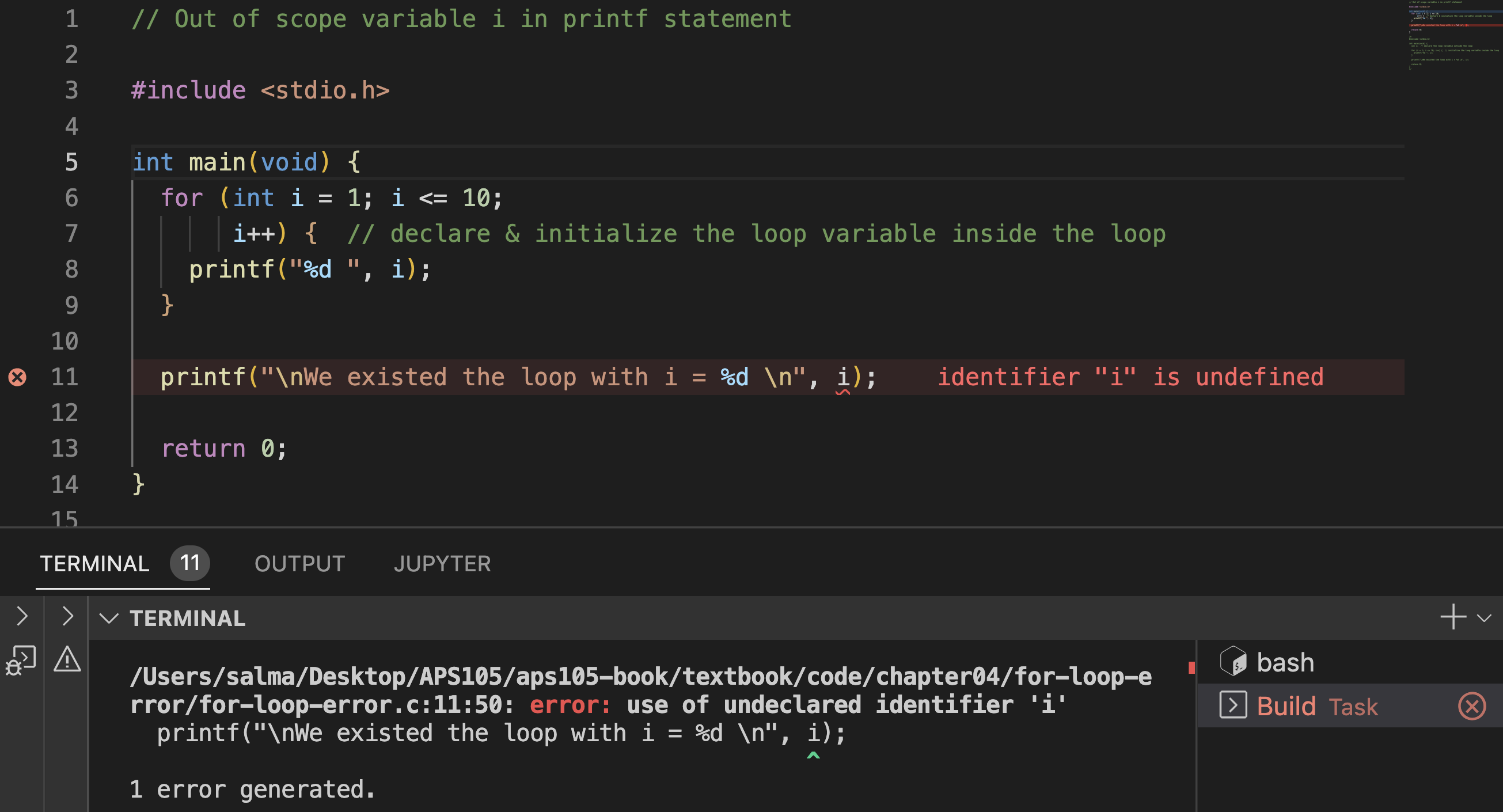
Fig. 4.10 The error message when the loop variable is used outside the loop.#
Solution! If you want to use the loop variable outside the loop, you can declare it outside the loop and initialize it inside the loop. For example, the following code will compile and run without any error. Download for-loop-scope.c
if you want to run the program yourself.
#include <stdio.h>
int main(void) { int i; // declare the loop variable outside the loop for (i = 1; i <= 10; i++) { // initialize the loop variable inside the loop printf("%d ", i); }
printf("\nWe exited the loop with i = %d \n", i);
return 0; }
4.3.3. Variations in for loop#
You can use the for loop in different ways, including omitting some statements.
You can omit the initialization and/or increment statements. For example, the following code snippet shows a for loop to print out the numbers \(1\) through \(10\) without initialization and increment statements.
1int i = 1; // initialization 2for (; i <= 10;) { 3 printf("%d ", i); 4 i++; // increment 5}
If the condition in the for loop is empty, then the default is that the condition is
true
. For example, the following code snippet with infinitely print*
.1for (;;) { 2 printf("*"); 3}
You can have more complex expressions in initialization or increment statements. For example, you can have multiple statements in the initialization or increment statements. Multiple statements should be separated by a comma
,
. If there were no statements in the body of the loop, the for loop should end with a;
. For example, the following code snippet will print out the numbers \(1\) through \(3\).for (int i = 1; i <= 3; printf("%d ", i), i++) ;
The order of execution for such for loop is shown in the following figure.
Fig. 4.11 The order of execution for a compact for loop.#
You can declare and initialize multiple variables in the initialization statement. For example, the following code snippet will print out the timetable of 7.
Code
#include <stdio.h>
int main(void) { for (int i = 1, j = 7; i <= 10; printf("7 * %d = %d\n", i, j), i += 1, j += 7) ; return 0; }You can have complex conditions. The condition can be complex using logical (
&&
,||
,!
) and relational operators (>
,>=
,<
,<=
,==
,!=
). Conditions cannot be separated using,
as the initialization and increment statements can.Exercise
Write a C program to print out the squares of the numbers from \(1\) to \(50\) or until the square of the number is greater than \(200\).
Step 1: Toy example. The expected output is
1 4 9 16 25 36 49 64 81 100 121 144 169 196
Step 2: Think of a solution! You can use a for loop to loop over the numbers from \(1\) to \(50\) and print their squares. However, there is an additional condition that the square of the number should be less than \(200\). You can add this condition statement to exit the loop when the condition is not satisfied.
Step 3: Decompose into steps. You can decompose the problem into the following steps.
Initialize a number to \(1\).
Print the square of the number.
Increment the number by \(1\).
Check if the square of the number is less than \(200\) and the number is less than \(50\).
If the condition is satisfied, go to step 2. Otherwise, exit the loop.
Step 4: Draw your solution (optional). You can draw your solution to help you write the correct code. The following figure shows the solution.
Fig. 4.12 The flow chart for the solution.#
Step 5: Write the code. You can write the code as follows.
Code
#include <stdio.h>
int main(void) { for (int num = 1; (num <= 50) && (num * num < 200); num += 1) { printf("%d ", num * num); } return 0; }Step 6: Test your code. You can test your code easily by looking at the numbers printed out. 196, which is the last square printed is less than 200. All numbers printed are squares. 196 is a square of 14, which is less than 50. Therefore, the code is \(\sim 100\%\) correct. I have the \(\sim\) to encourage you to be skeptical!
4.3.4. How to choose the kind of the loop: while, do-while, for?#
You can use the:
for
: if you know the number of iterations in advance.do-while
: if you want to test the condition after executing the body of the loop at least once.while
: if you want to test the condition before executing the body of the loop.
Quiz
0 Questions