Nested-if statements
Contents
3.3. Nested-if statements#
In the previous section, we developed the following program that printed to the user if they’re eligible to work in Ontario, Canada based on their age. We wrote an if-else
statement inside the else
of another if-statement. This is referred to as a nested-if statement. Nested-if statements are useful when we want to check multiple conditions as we observed in the previous section.
Not elegant code
#include <stdio.h> int main(void) { int age = 0; printf("Enter your age: "); scanf("%d", &age); if (age < 14) { printf("You are not yet eligible to work."); } else { // Note! age >= 14 is redundant, in the following condition // since the else block will be executed only if age >= 14 if (age >= 14 && age <= 16) { printf("You are eligible to work only outside school hours."); } else { printf("You are eligible to work."); } } return 0; }
In the following code, we wrote a more elegant code. In line 9, we can see else if (<condition>)
, which is evaluated only if the condition in the preceding if
statement is false
. This is also called a nested-if statement, but it is more readable and elegant.
More elegant code
#include <stdio.h> int main(void) { int age = 0; printf("Enter your age: "); scanf("%d", &age);
if (age < 14) { printf("You are not yet eligible to work in Ontario."); } else if (age >= 14 && age <= 16) { // Can omit age >=14, since it is redundant printf("You are eligible to work only outside school hours."); } else { printf("You are eligible to work in Ontario."); } return 0; }
3.3.1. Longer nested-if statements#
Let’s develop a C program that finds the maximum of three integers x
, y
and z
and stores the maximum int in a variable named max
.
Step 1: Toy example. Let’s say we have x = 3
, y = 4
and z = 5
. The maximum is expected to be z
.
Step 2: Think of a solution. We can compare two integers first, and then the maximum of them can be compared with the third int. For example, we compare x
and y
, and the larger number gets compared with z
.
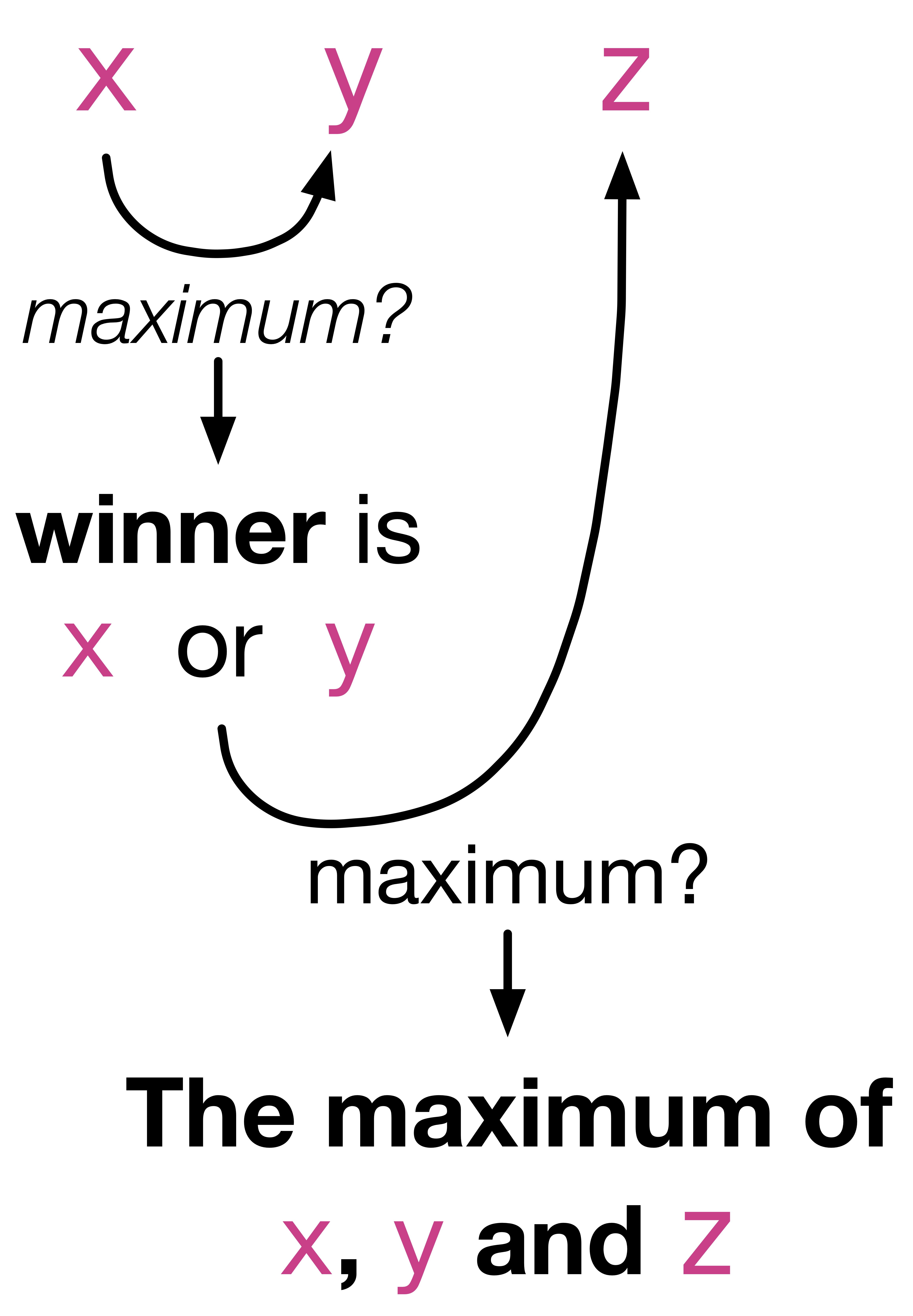
Fig. 3.4 The steps of comparing three numbers to find the maximum.#
Step 3: Decompose solution into steps. First, we compare x
and y
. If x
is larger, we compare x
and z
. If y
is larger, we compare y
and z
. Second, we compare the maximum of x
and y
with z
. If the maximum is larger, we print the maximum. If z
is larger, we print z
.
Step 4: Draw your solution. The following flow chart shows the steps of the solution.
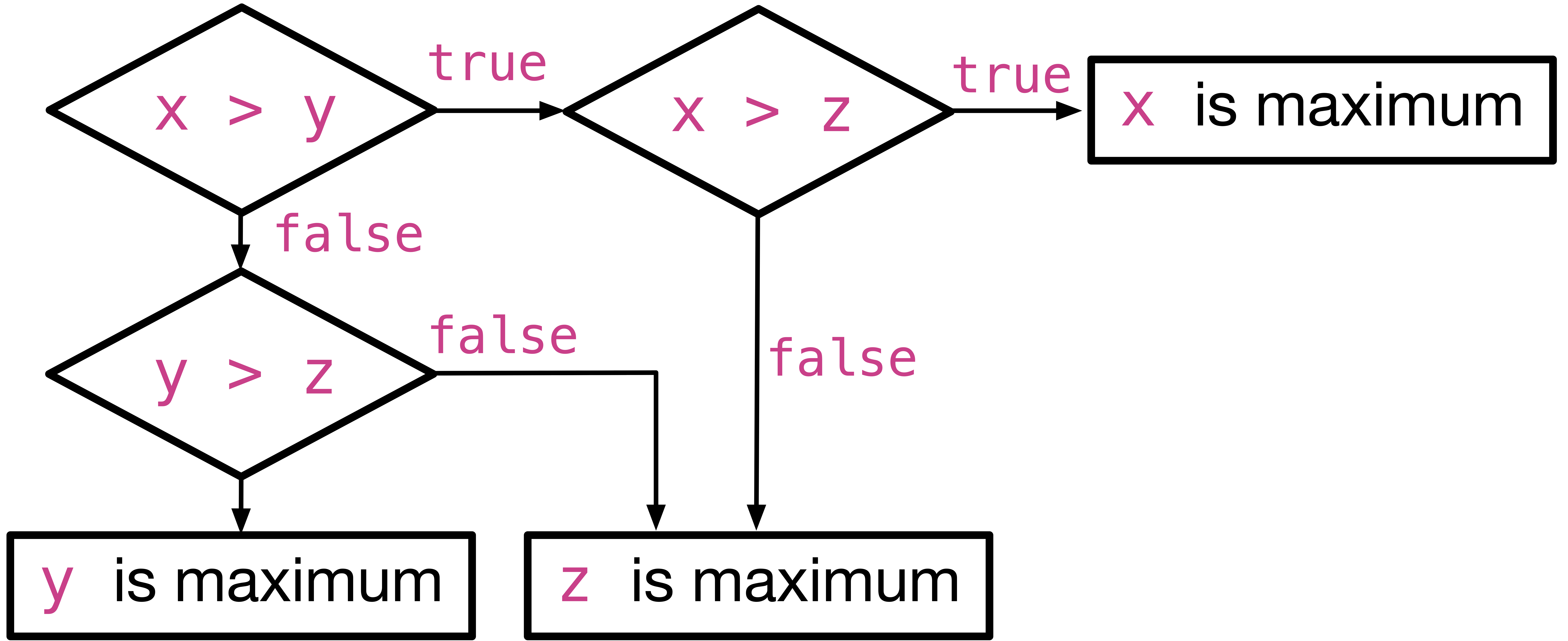
Fig. 3.5 Flow chart of the steps of comparing three numbers to find the maximum.#
Step 5: Make sure your steps works on other toy examples. Try a toy example with the maximum number in a different variable. For example, x = 5
, y = 3
and z = 4
. The maximum is expected to be x
.
Step 6: Write the code. The following code implements the steps of the flow chart. Download max-of-three-method1.c
to get the following code.
Code
#include <stdio.h> int main(void) { int x = 0, y = 0, z = 0; printf("Enter three integers: "); scanf("%d %d %d", &x, &y, &z);
// Maximum of x and y if (x > y) { if (x > z) { printf("The maximum is the first number entered: %d", x); } else { printf("The maximum is the third number entered: %d", z); } } else { if (y > z) { printf("The maximum is the second number entered: %d", y); } else { printf("The maximum is the third number entered: %d", z); } } return 0; }
More elegant code can be written if we thought backwards. We can think of the condition that is true
only if x
is the maximum, i.e., (x > y && x > z)
and the conditions that is true only if y
is the maximum, i.e., (y > x && y > z)
and else
z
is the maximum. The following code implements this idea. Download max-of-three-method2.c
Code
#include <stdio.h> int main(void) { int x = 0, y = 0, z = 0; printf("Enter three integers: "); scanf("%d %d %d", &x, &y, &z);
if (x > y && x > z) { printf("The maximum is the first number entered: %d", x); } else if (y > x && y > z) { printf("The maximum is the second number entered: %d", y); } else { printf("The maximum is the third number entered: %d", z); } return 0; }
3.3.2. Dangling else problem#
The brackets {}
in the if-statements are not necessary when the code block is one line. For example, the following code is valid without brackets {}.
Code without brackets {}
if (<condition>)
<statement>;
else
<statement>;
However, it is a good practice to use brackets {}
to avoid an issue referred to as the dangling else problem. For example, in the following code, the else
belongs to which if
statement? By default, the else
belongs to the if
statement that is closest to it, if there were no brackets.
Code with dangling else problem
if (<condition>)
if (<condition>)
<statement>;
else // this else belongs to the if statement above
<statement>;
Quiz
0 Questions